Home / IT & Computer Science / AI & Robotics / Get ready for a Masters in Data Science and AI / Conditional expressions
This article is from the free online
Get ready for a Masters in Data Science and AI
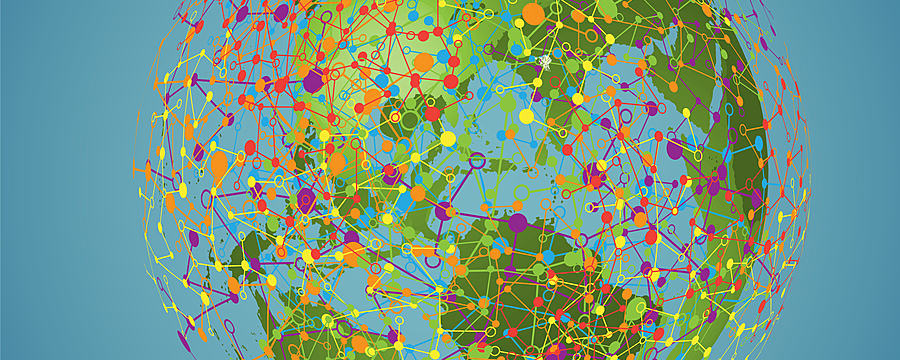
Reach your personal and professional goals
Unlock access to hundreds of expert online courses and degrees from top universities and educators to gain accredited qualifications and professional CV-building certificates.
Join over 18 million learners to launch, switch or build upon your career, all at your own pace, across a wide range of topic areas.